This Node.js Trick Feels Illegal… But It Works 🤯 | Cluster Module for Noobs
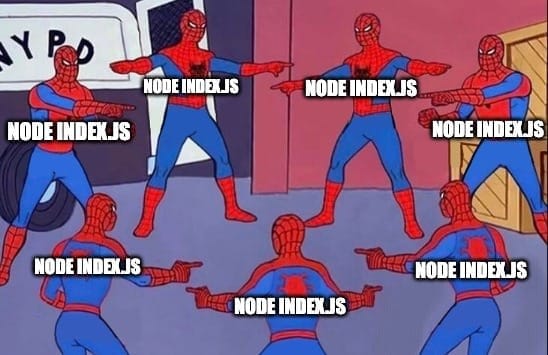
Ever wondered why your Node.js server is chilling on one CPU core while the other cores are just vibing?
Good news: You’ve stumbled upon a "feels-illegal-but-it’s-not" trick to unleash the true power of Node.js using the cluster module.
Let’s dive into how this mysterious module turns your single-threaded server into a multi-core monster
But Wait… Isn’t Node.js Single-Threaded?
Yes. Node.js runs on a single thread. That means even if you have a 16-core beast of a CPU, your app is stuck using just one of them by default.
And that’s where the Cluster module comes in — it lets you spawn multiple instances of your app across all available CPU cores.
What’s the Cluster Module?
The cluster module is built into Node.js. It allows you to create child processes (workers) that all share the same server port.
Each worker runs on its own event loop, which means… parallelism, baby.
Let’s See It in Action
Here’s a basic example:
const cluster = require('cluster');
const http = require('http');
const os = require('os');
const numCPUs = os.cpus().length;
if (cluster.isPrimary) {
console.log(`Primary process ${process.pid} is running`);
// Fork workers
for (let i = 0; i < numCPUs; i++) {
cluster.fork();
}
// Optional: Log when workers die
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
cluster.fork(); // Restart a worker if it dies
});
} else {
// Workers can share any TCP connection
http.createServer((req, res) => {
res.writeHead(200);
res.end(`Hello from Worker ${process.pid}\n`);
}).listen(3000);
console.log(`Worker ${process.pid} started`);
}
What Just Happened?
cluster.isPrimary
: Checks if the current process is the master process.cluster.fork()
: Spawns a new worker process.- Each worker runs its own copy of the HTTP server.
- All workers share the same port (
3000
) and load is balanced automatically.
How I Use It
To better manage how my app runs across multiple cores, I separate the server logic from the clustering logic. Instead of running my app directly in server.js
, I create a separate cluster.js
file that manages the clustering, while server.js
simply exports the app logic.
This allows me to use Node's cluster
module to spawn multiple instances of my app and distribute the load across all available CPU cores.
Here’s a step-by-step breakdown:
Step 1: Create the server.js
File
In server.js
, we define the basic app behavior. For example, here’s a simple HTTP server:
// server.js
const http = require('http');
// Create a simple HTTP server that responds with "Hello, World!"
const app = http.createServer((req, res) => {
res.writeHead(200);
res.end('Hello, World!');
});
// Export the app to be used by another module
module.exports = app;
In this file, we just define the basic behavior of the app (in this case, a simple HTTP server). By exporting the app, we make it reusable in other files.
Step 2: Create the cluster.js
File
Now, in cluster.js
, we’ll use the cluster
module to spawn multiple instances of our app. The cluster.isPrimary
condition checks if the current process is the master process, and if it is, it will spawn worker processes to handle the load.
// cluster.js
const cluster = require('cluster');
const os = require('os');
const app = require('./server'); // Import the app from server.js
const numCPUs = os.cpus().length; // Get the number of CPU cores available
if (cluster.isPrimary) {
console.log(`Primary process ${process.pid} is running`);
// Fork workers, one for each CPU core
for (let i = 0; i < numCPUs; i++) {
cluster.fork(); // Creates a worker process for each core
}
// Optional: Log when workers die
cluster.on('exit', (worker, code, signal) => {
console.log(`Worker ${worker.process.pid} died`);
cluster.fork(); // Restart a worker if it dies
});
} else {
// Workers run the server
app.listen(3000, () => {
console.log(`Worker ${process.pid} is running`);
});
}
How It Works
cluster.isPrimary
: Checks if the current process is the master (primary) process. If it is, it forks multiple worker processes usingcluster.fork()
.cluster.fork()
: This spawns a new worker for each available CPU core. Each worker gets its own event loop, allowing them to handle requests independently.- Workers share the same port (
3000
in this case), and the load is automatically distributed across them. - If a worker crashes, it’s restarted automatically, ensuring the server remains highly available.
Step 3: Run the Clustered App
To run the clustered version of your app, simply execute cluster.js
:
node cluster.js
Now, instead of having a single instance of your app running, you’ll have one worker process for each available CPU core. This allows your app to handle more traffic, as each core can handle requests independently.
Why This Approach?
By separating the server logic (server.js
) from the clustering logic (cluster.js
), we can:
- Keep the server code clean and focused on the application logic.
- Easily manage the clustering behavior in one dedicated file.
- Maintain the ability to scale the app across multiple cores with minimal effort.
Why Bother With This?
- Use all CPU cores instead of one.
- Improve performance under high load.
- More resilient — if one worker crashes, others keep running.
- Easy horizontal scaling (combine with PM2, Docker, etc.).
But There Are Caveats
- Memory isn’t shared across workers — they don’t talk to each other by default.
- You’ll need IPC (inter-process communication) or a shared cache (like Redis) for shared data.
- Doesn’t scale across multiple machines — just cores on a single one.
Pro Tips
- Use a process manager like PM2 for production use — it handles clustering, logging, and restarts.
- Monitor worker crashes — don’t let your server die silently.
- If your app is CPU-bound (e.g., image processing), combine clusters with worker threads.
Final Thoughts
The cluster
module is like the secret turbo boost for Node.js apps — especially for CPU-heavy or high-traffic servers.
You’re still writing the same Node.js code, but now your app is scaling like a pro across multiple cores.
So yeah… it might feel illegal — but it’s 100% built into Node.js and totally fair game. Now go melt some CPUs (safely)